Unraveling the For Loop: A Pillar of Programming
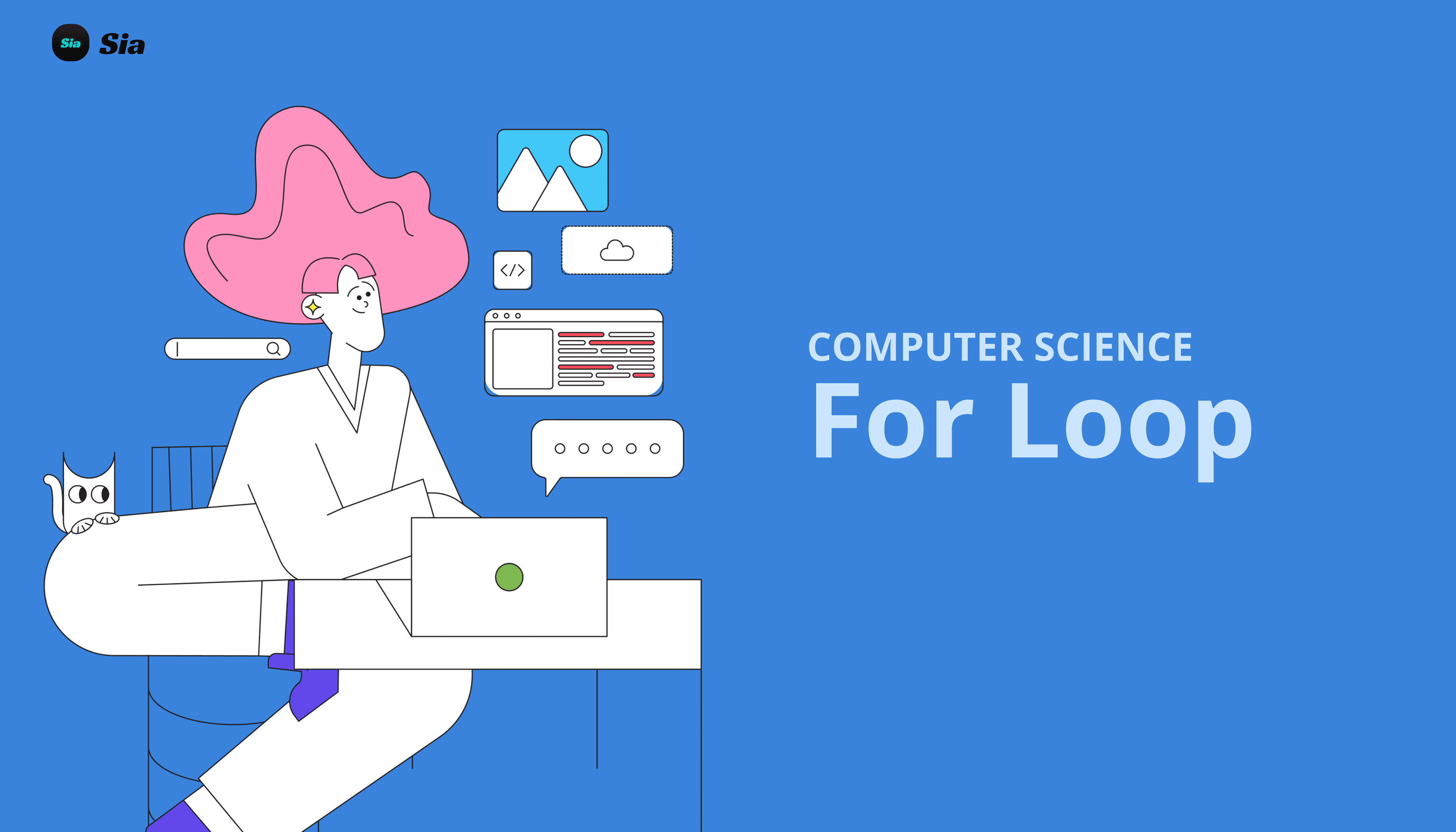
Student Question: Tell me the concept of for loop
Sia Response:
The concept of a for loop in programming is a control flow statement that allows code to be executed repeatedly based on a given boolean condition. The for loop is used to iterate over a sequence (that could be a list, a tuple, a dictionary, a set, or a string). With the for loop, we can execute a set of statements, once for each item in a list, tuple, set, etc.
Here is a basic example of a for loop in Python:
for i in range(5):
print("Hello World", i)
The above code will print "Hello World" followed by the current value of i, five times, starting from 0 to 4.
Structure of a For Loop:
-
Initialization: The loop starts with an initialization of the iterator.
-
Condition: It is followed by a condition that is evaluated before each iteration. If the condition is true, the code block within the loop is executed.
-
Iteration: After the code block is executed, the iterator is updated.
-
Termination: The loop terminates when the condition becomes false.
The for loop is a fundamental concept in computer science that is used in various applications such as iterating through data structures, implementing algorithms, and automating repetitive tasks.
Learn smart through AskSia: Learn More